Blogger Live Search Plugin with jQuery and JavaScript
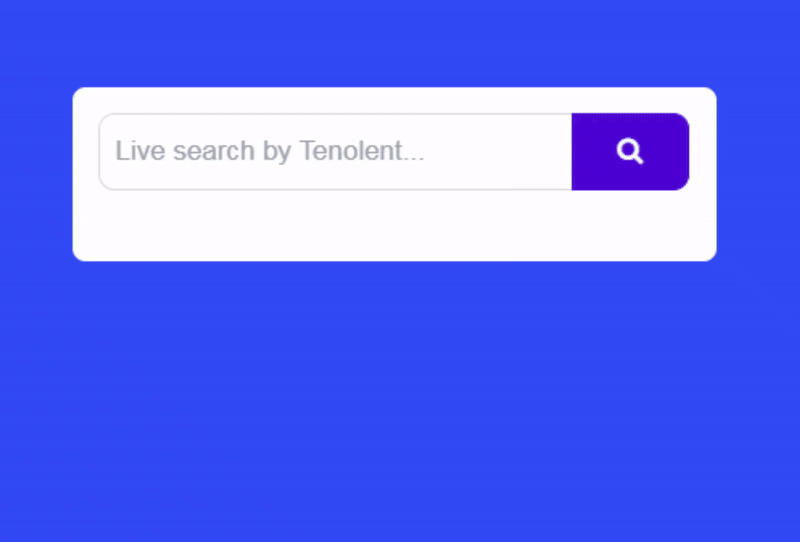
Live search is an essential feature for modern websites, allowing users to find the content they’re looking for instantly without reloading the page. For Blogger users, implementing a live search functionality can significantly enhance user experience. In this article, we’ll guide you through creating a Blogger Live Search Plugin using jQuery.
Why Live Search?
A live search plugin improves the efficiency and usability of your website by:
- Enhancing User Experience: Visitors can see search results in real-time without navigating to a new page.
- Saving Time: Instant feedback reduces the time spent searching for content.
- itImproving Engagement: Quick access to relevant posts keeps users engaged.
Features of the Plugin
- Real-time search results.
- Works seamlessly with Blogger’s JSON API.
- Customizable design and animations.
- Lightweight and efficient.
Prerequisites
Before getting started, ensure that:
- You have a Blogger blog.
- You include the jQuery library in your template. Add this script to your Blogger theme header:
<!--Jquery CDN: Live Search by Tenolent-->
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
<script src="https://cdn.tailwindcss.com"></script>
Step-by-Step Implementation
1. HTML Structure
Add a search bar and a results container to your Blogger template or widget:
<!--HTML Codes: Live Search by Tenolent-->
<div class="live-search mx-auto max-w-[400px] bg-white p-4 rounded-lg">
<form class="live-search-form" action="/search">
<input autocomplete="off" type="search" id="search-input" name="q" placeholder="Live search by Tenolent..." required="" value="" />
<button type="submit">
Search
</button>
</form>
<span class="flex items-center justify-center mt-2">
<span class="text-gray-700 text-sm" id="search-spinner" aria-hidden="true" style="display: none;">Loading...</span>
</span>
<div class="mx-0 bg-white px-2 pb-4 pt-3 rounded-md" id="search-results"></div>
</div>
2. CSS Styling
Style the search bar and results for a polished look:
/**CSS Codes: Live Search by Tenolent**/
form.live-search-form input[type=search] {
padding: 10px;
font-size: 16px;
border-left: 1px solid #ddd;
border-bottom: 1px solid #ddd;
border-top: 1px solid #ddd;
border-radius: 10px 0px 0px 10px;
float: left;
width: 80%;
background: #fff;
}
form.live-search-form input[type=search]:focus {
outline: none;
}
form.live-search-form button {
float: left;
width: 20%;
padding: 11px 10px;
background: #5115dd;
color: white;
font-size: 15px;
border-right: 1px solid #5115dd;
border-top: 1px solid #5115dd;
border-bottom: 1px solid #5115dd;
border-radius: 0px 10px 10px 0px;
cursor: pointer;
}
form.live-search-form button:hover {
background: #5118ee;
}
form.live-search-form::after {
content: "";
clear: both;
display: table;
}
input:-webkit-autofill,
input:-webkit-autofill:hover,
input:-webkit-autofill:focus,
input:-webkit-autofill:active{
-webkit-box-shadow: 0 0 0 30px white inset !important;
}
3. jQuery Script
Use the following jQuery script to fetch search results from Blogger’s JSON API:
<script>
// JS Codes: Live Search by Tenolent
function search() {
const query = $("#search-input").val().trim().toLowerCase();
if (query.length < 2) return $("#search-results").empty();
$("#search-spinner").show();
$.ajax({
url: "/feeds/posts/summary?alt=json-in-script&callback=?&max-results=1000",
dataType: "jsonp",
success: function(data) {
const results = data.feed.entry || [];
const filteredResults = results.filter(post => post.title.$t.toLowerCase().includes(query));
const count = filteredResults.length;
if (count) {
const foundText = `<div class="mt-0 mb-4 text-left italic text-gray-500">
We Found <span>${count}</span> results matching the keywords <span class='font-bold'>${query}</span>
</div>`;
const postItems = filteredResults.slice(0, 4).map(post => {
const title = post.title.$t;
const url = post.link.find(link => link.rel === "alternate").href;
return `<div class="py-3" style="border-bottom: 1px solid #ddd;">
<a href="${url}" class="block text-md font-medium text-black">
${title}
</a>
</div>`;
}).join("");
const viewAllButton = `<a href="/search?q=${query}">
<button class="bg-black text-white font-medium rounded px-6 py-3 mt-3">
View all results
</button>
</a>`;
$("#search-results").html(foundText + postItems + viewAllButton);
} else {
$("#search-results").html(`<div class="mt-12 text-center">
<span class="font-bold text-3xl">No results found.</span>
<span class="block">Try a different keyword.</span>
</div>`);
}
$("#search-spinner").hide();
}
});
}
$(document).ready(function() {
$("#search-input").on("keyup", search);
});
</script>
4. Save and Test
After adding the code, save your changes in the Blogger theme editor and test the live search functionality. Type keywords into the search bar and see the results populate in real-time.
Advanced Customizations
Conclusion
Implementing a live search plugin in your Blogger website using jQuery is straightforward and highly beneficial for improving user experience. With just a few lines of code, you can provide real-time search functionality to your visitors. Customize the design and behavior to suit your blog’s style, and watch as your website becomes more interactive and user-friendly!
Example
Comments
Post a Comment